How to: Use the SharePoint 2013 REST API from a Windows Store App (Windows 8 Metro)
Posted
Friday, September 28, 2012 9:52 AM
by
CoreyRoth
I’ve recently taken an interest to building Windows Store Apps (aka Windows 8 Metro). Not because I think I can get rich in the Windows Store but because I think it provides a unique way to present SharePoint data. If you’re like me, you probably assumed Windows Store App development was just like .NET Framework 4.5 development. You would be wrong. The .NET Framework is provided by a single assembly reference called .NET for Windows Store Apps. It has many of the familiar classes that you have come to expect. My initial thinking was that I could use the SharePoint Client Object Model directly from my app. That thinking was also wrong. It turns out that the Client Object Model has a dependency on System.Web.Service.dll which does not exist in .NET for Windows Store Apps. Simply put, Window Store Apps only support WCF references. This means that the REST API is now our best choice.
When it comes to Windows Store Apps, you have a few choices on which programming language to use. Modern JavaScript is not my strong point, so I am going to demonstrate using C#. Before we dig into the C# code though, we’ll take a look at how to construct the REST URL. This article on MSDN does a great job explaining the details of how the URL is built. Essentially we start with the URL to our site (i.e.: http://server/site) and append /_api/web/lists to it along with a method to get a particular list by title, getbytitle. To get the items of the list we append /items to it. In my case, I want to pull the tasks list of a site so it would look something like this.
http://server/site/_api/web/list/getbytitle(‘tasks’)/items
You can type this right into your browser and see the XML that the REST API returns. Here’s an example.
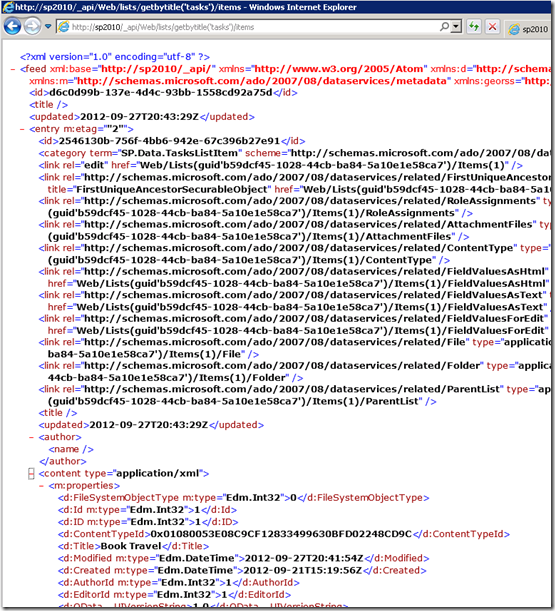
There is a lot of XML to take in there. We’re particularly interested in the content element contain in each entry element. This has the data from our list items. However, we can reduce the amount of XML returned significantly by using the $select parameter on the REST URL. In my case I am going to select a few common fields such as Title, DueDate, and Status. Here is what the URL looks like now.
http://server/site/_api/web/list/getbytitle(‘tasks’)/items?$select=Title,DueDate,Status,PercentComplete
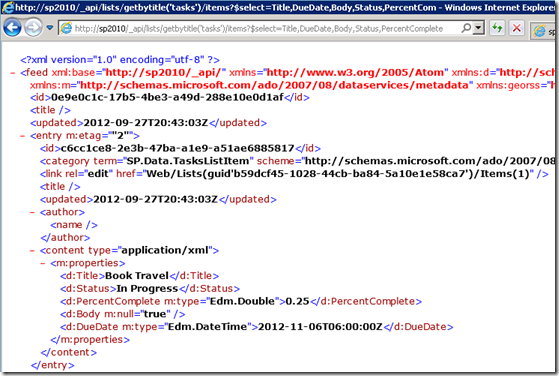
You’ll notice that the results are much more manageable now. We’ll talk about how we can use LINQ to XML here shortly to parse this data into something usable from our Windows Store app.
Now let’s look at the code that is required to get the data in our app. Start by creating a new Windows Store App in Visual Studio 2012. You can do this by choosing your language (C#) and then Windows Store –> Blank App (XAML).
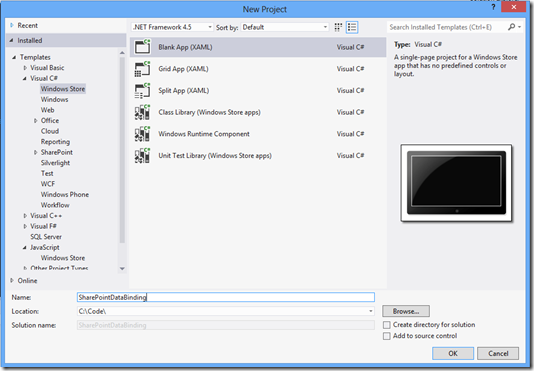
If this is the first Windows Store App you have started in Visual Studio, you will be prompted for your Windows Live credentials. This allows you to get a certificate so that you can build apps locally. In our app, we want to bind data, so I am going to create a new Items Page. This template has many of the components you need to get started quickly with data binding.
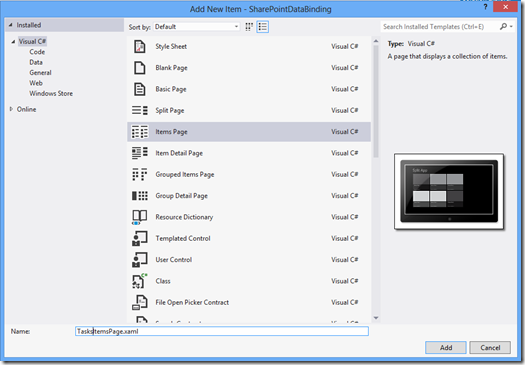
When adding the page, you will be prompted to add some dependent files. Click yes and then you should see your design surface. Now, open the code-behind of your Items page. In the LoadState method, we will start the process of retrieving our data and binding it. We rely on some asynchronous calls to get data from REST, so we will need to put this data in another method and use the async keyword.
protected override void LoadState(Object navigationParameter, Dictionary<String, Object> pageState)
{
// TODO: Assign a bindable collection of items to this.DefaultViewModel["Items"]
BindData();
}
private async void BindData()
{
}
Before we start writing the method though, we need to include a few using statements.
using System.Net;
using System.Net.Http;
using System.Xml;
using System.Xml.Linq;
If you have looked at any of the other C# examples for working with the REST API, you will know they are heavily dependent on the HttpWebRequest object. However, with Windows Store Apps, we have to use HttpClient instead. We’ll start by defining our REST URL from up above.
string restUrl = "http://server/_api/lists/getbytitle('tasks')/items?$select=Title,DueDate,Body,Status,PercentComplete";
To handle authentication we first need to create an HttpClientHandler object. We want to automatically use the credentials of the currently logged in user so this is required. To enable this, just set UseDefaultCredentials to true. There are also other settings required which we’ll talk about below.
HttpClientHandler httpClientHandler = new HttpClientHandler();
httpClientHandler.UseDefaultCredentials = true;
We can now create the HttpClient object by passing the HttpClientHandler to the constructor.
HttpClient client = new HttpClient(httpClientHandler);
We then need to tell the REST API, that we want the result back as ATOM / XML as opposed to JSON. We do this by setting a few headers.
client.DefaultRequestHeaders.Add("Accept", "application/atom+xml");
client.DefaultRequestHeaders.Add("ContentType", "application/atom+xml;type=entry");
Now, we can send the request to SharePoint and wait for a response. We use the await keyword on the GetAsync() method which is why we needed the async keyword on the method signature. The EnsureSuccessStatusCode() method simply throws an exception if a valid 200 HTTP response is not received.
var response = await client.GetAsync(restUrl);
response.EnsureSuccessStatusCode();
At this point our data has been received back at the client from SharePoint. Now we need to begin the fun process of parsing it and binding it. We get the raw string XML data with the following method (also asynchronous). You could run the app at this point (with a few tweaks) if you wanted to see it in the debugger but we haven’t done anything wit the data yet.
string responseBody = await response.Content.ReadAsStringAsync();
Now we need to read the XML into an XDocument object so that we can use LINQ to XML to parse it.
StringReader reader = new StringReader(responseBody);
XDocument responseXml = XDocument.Load(reader, LoadOptions.None);
Now we have the data into something queryable. However, what we really need is a custom object that we can bind to. If you look back at the XML, you might have noticed a few namespaces in use in the XML document. We need to define those or our queries will never work.
XNamespace atom = "http://www.w3.org/2005/Atom";
XNamespace d = "http://schemas.microsoft.com/ado/2007/08/dataservices";
XNamespace m = "http://schemas.microsoft.com/ado/2007/08/dataservices/metadata";
Now, we can use LINQ to XML to select the data into a new anonymous type. If you look at the XML again, we want to query entry elements using the Descendants() method and then the actual values we want are inside the nested Content and then Properties elements. The data is heavily nested so it makes our LINQ to XML a little messy. It’s not too bad though. For the non-string values, I parse the data into the type that I want it (i.e.: DateTime).
var items = from item in responseXml.Descendants(atom + "entry")
select new
{
Title = item.Element(atom + "content").Element(m + "properties").Element(d + "Title").Value,
DueDate = DateTime.Parse(item.Element(atom + "content").Element(m + "properties").Element(d + "DueDate").Value),
Status = item.Element(atom + "content").Element(m + "properties").Element(d + "Status").Value,
PercentComplete = decimal.Parse(item.Element(atom + "content").Element(m + "properties").Element(d + "PercentComplete").Value)
};
Now, we have data that can be bound to the GridView / ListView on the Items Page. To bind we assign our data source as follows:
this
.DefaultViewModel["Items"] = items; Just like Windows Phone and SharePoint apps need to declare the capabilities of the application, Windows Store Apps are no different. To do this, open Package.appmanifest. In our case, we need to declare that we intend to communicate over the internal network (Private Networks) and that we need Enterprise Authentication. The latter allows the app to authenticate with the current user’s domain credentials automatically. If you don’t have this capability set, you are guaranteed a 401 Access Denied or Unable to connect to remote server error.
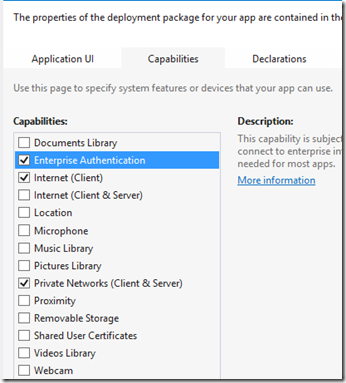
We can now run the app. However, there is one last thing we need to do. We need a way to navigate to the TaskItemsPage that we created. It loads MainPage by default. Without getting to deep into how Windows Store app navigation works, we’ll just throw a button on the MainPage and then navigate to the TaskItemsPage when clicked. You can drag and drop a button onto the MainPage using the designer. Double click on it and it will create the event handling method. Then we use Frame.Navigate() and pass it the type of page we want to go to, TaskItemsPage. Here is the code.
private void Button_Click_1(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(TasksItemsPage));
}
Run your app, and click on the button to navigate to your TaskItemsPage. If all goes well, you won’t receive any errors and you’ll see a page like the screen below. Note, that your application doesn’t currently have any way to exit. To get out you either need to Alt+Tab or move your mouse to the Top-Left corner of the screen to switch to the desktop.
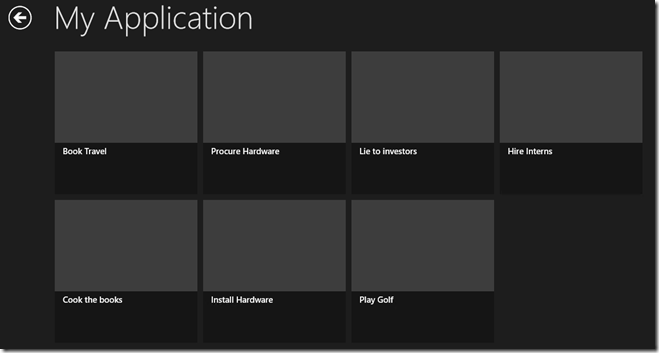
This is great, but we’re not using all of our data yet. By default, it will bind the Title for us. However, we want to display some of our additional fields though. To do this, we can create a DataTemplate. We need to look into how it works first though. Locate the GridView in your XAML file. It should look something like this.
<GridView
x:Name="itemGridView"
AutomationProperties.AutomationId="ItemsGridView"
AutomationProperties.Name="Items"
TabIndex="1"
Grid.RowSpan="2"
Padding="116,136,116,46"
ItemsSource="{Binding Source={StaticResource itemsViewSource}}"
ItemTemplate="{StaticResource Standard250x250ItemTemplate}"
SelectionMode="None"
IsSwipeEnabled="false"/>
Notice the ItemTemplate and how it is bound to Standard250x250ItemTemplate. This template can actually be found in StandardStyles.xaml.
<DataTemplate x:Key="Standard250x250ItemTemplate">
<Grid HorizontalAlignment="Left" Width="250" Height="250">
<Border Background="{StaticResource ListViewItemPlaceholderBackgroundThemeBrush}">
<Image Source="{Binding Image}" Stretch="UniformToFill" AutomationProperties.Name="{Binding Title}"/>
</Border>
<StackPanel VerticalAlignment="Bottom" Background="{StaticResource ListViewItemOverlayBackgroundThemeBrush}">
<TextBlock Text="{Binding Title}" Foreground="{StaticResource ListViewItemOverlayForegroundThemeBrush}" Style="{StaticResource TitleTextStyle}" Height="60" Margin="15,0,15,0"/>
<TextBlock Text="{Binding Subtitle}" Foreground="{StaticResource ListViewItemOverlaySecondaryForegroundThemeBrush}" Style="{StaticResource CaptionTextStyle}" TextWrapping="NoWrap" Margin="15,0,15,10"/>
</StackPanel>
</Grid>
</DataTemplate>
If you look at what it is binding, it’s looking for fields Image, Title, and Subtitle. We don’t have an image, so we can get rid of that. In its place, we’ll add the other fields in our dataset. We don’t want to edit the out-of-the-box template, so we can copy this snippet and add it to the Pages.Resources element in our TaskItemsPage.xaml. I then changed its name to TaskItemTemplate and added the remaining fields.
<DataTemplate x:Key="TaskItemTemplate">
<Grid HorizontalAlignment="Left" Width="250" Height="250">
<StackPanel VerticalAlignment="Top" Background="{StaticResource ListViewItemOverlayBackgroundThemeBrush}">
<TextBlock Text="{Binding Title}" Foreground="{StaticResource ListViewItemOverlayForegroundThemeBrush}" Style="{StaticResource TitleTextStyle}" Height="60" Margin="15,0,15,0"/>
<TextBlock Text="{Binding DueDate}" Foreground="{StaticResource ListViewItemOverlaySecondaryForegroundThemeBrush}" Style="{StaticResource CaptionTextStyle}" TextWrapping="NoWrap" Margin="15,0,15,10"/>
<TextBlock Text="{Binding PercentComplete}" Foreground="{StaticResource ListViewItemOverlaySecondaryForegroundThemeBrush}" Style="{StaticResource CaptionTextStyle}" TextWrapping="NoWrap" Margin="15,0,15,10"/>
<TextBlock Text="{Binding Status}" Foreground="{StaticResource ListViewItemOverlaySecondaryForegroundThemeBrush}" Style="{StaticResource CaptionTextStyle}" TextWrapping="NoWrap" Margin="15,0,15,10"/>
</StackPanel>
</Grid>
</DataTemplate>
Now we just need to change the template name in the GridView to use the TaskItemTemplate.
<GridView
x:Name="itemGridView"
AutomationProperties.AutomationId="ItemsGridView"
AutomationProperties.Name="Items"
TabIndex="1"
Grid.RowSpan="2"
Padding="116,136,116,46"
ItemsSource="{Binding Source={StaticResource itemsViewSource}}"
ItemTemplate="{StaticResource TaskItemTemplate}"
SelectionMode="None"
IsSwipeEnabled="false"/>
Run the app again and you’ll now have your custom fields bound. It should look something like this.
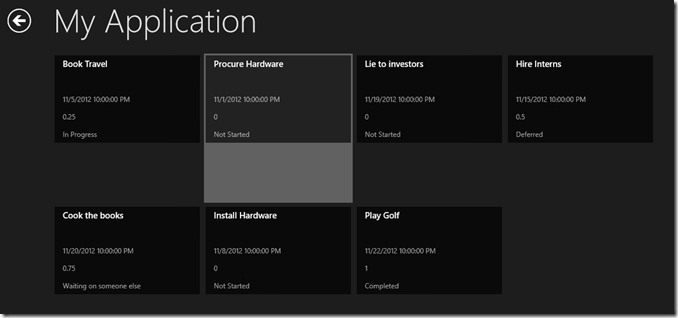
The DueDate and PercentComplete are not formatted in this case. I won’t cover that today, but if you look at the Blog Reader example, it explains the techniques you can use to do cool formatting. The Data Binding article goes into a lot of detail if you want to learn all of the ways you can bind data too. If you want a good tutorial to start from scratch on a Windows Store App, see the Hello, World example.
This turned out to be quite the long blog post. I hope you find it useful. If you find this interesting and you are going to be at SharePoint Conference 2012 in November (SPC12), you should be sure and attend my session Bringing SharePoint to the Desktop: Building Windows Store Apps with SharePoint 2013 (SPC025).
Follow me on twitter @coreyroth.