Azure App Service makes it easy to build a back-end for a mobile application. One of my favorite features of it is Easy Tables. Easy Tables makes it easy to create tables in a SQL Azure database and comes with an SDK for several mobile platforms that make it easy to access it. You can make calls to it using REST, but there are APIs for Apache Cordova, Xamarin, as well as native development. Some of the APIs even support offline synchronization. If you are looking to prove out an idea, Easy Tables makes it well, easy.
There is some great documentation out there for getting started with Easy Tables as well. However, I ran into a few stumbling points in my early experiences that I thought I would share. These instructions assume, you have created an Azure App Service app. If you don’t have one just look for App Services in your Azure portal and click New. In our case, we are going to be working with a node.js back-end.
Setting up the database connection
Setting up the database connection should be simple, but unfortunately, it’s not due to issues in the configuration UI. Before you go to set up the connection, you will need to create a SQL Server to host your database if you don’t have one already. This isn’t terribly complicated just try to pick a region close to the one hosting your App Service App. After your database is set up, you need to create the database to host your data. You can use the free pricing tier too to try things out if you haven’t created a free database in this particular region yet (you only get one per region).
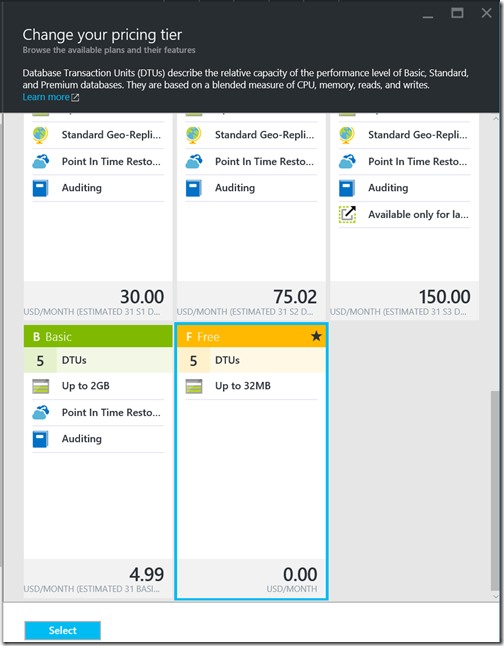
Once you have your database set up, you are ready to configure a connection to from Azure App Service. Effectively, you are creating a connection string. To set up the connection, go to your App Service App and click on the Easy Tables link under Mobile.
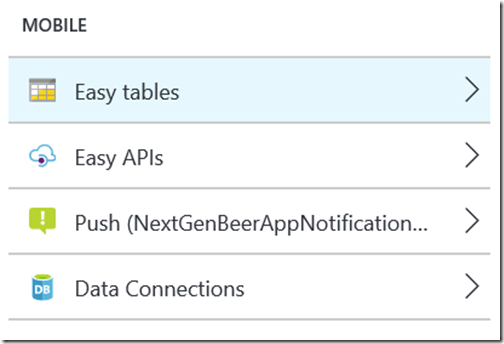
It will then initialize Easy Tables if needed and prompt you to click continue to set it up.
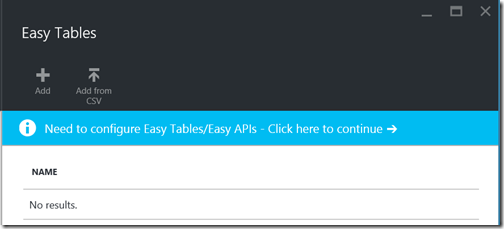
Here is where, it will prompt you to connect to a database.
After this, it will prompt you to select your existing SQL Server and then database. The final step is to enter in the username and password you set on your SQL Server and database. Specify a connection string name (whatever you want) along with the credentials. Click ok and hope for the best. I mentioned I had problems with the UI right? The first time I did this, I couldn’t get it to save my password because it had a semicolon (;) in it. Remember, how I said it is building a connection string underneath? Semicolon is a delimiter for connection strings and the UI doesn’t handle that at all. It just fails. Hopefully this step works for you. If it doesn’t there is a way that you can manually set the connection string using Resource Explorer. That’s more detail than I want to go into today. If you run into it though, feel free to ping me or leave me a comment and I’ll provide the details.
Once your database connection has been set up, click on the Initialize App button. This will destroy any files that may already exist on your App Service App so don’t click it if there is something you need there. Effectively this sets up folders such as /tables and /api as well as some of the basic node.js configuration. It also creates a test table called TodoItem.
Creating new tables
There are a few ways to create new tables but not all of them have the desired results:
- Create the appropriate files in the tables folder
- Create the table through the Azure Portal in the Easy Tables section
- Manually create the table in SQL Server
I would say the preferred way to create the table is by creating the appropriate files in the tables folder. The node.js back-end will create new SQL table for any .js file you add to the tables folder (assuming the appropriate supporting files are present). For example if you create a file named Event.js, you will get a SQL table called Event. If you create a file named Contact.js, you will get a SQL table called Contact. You get the idea. There is a little bit more to it though. Let’s look at the steps involved.
First, you need to be able to edit the files of your node.js App Service app. You have several ways to do this. I recommend setting up a local git repository and pushing files up to your App Service App. You can configure that along with credentials in the Deployment source setting of your App Service App. You can find more about setting up the node.js back-end and deployment in this article. However, you can also just edit the files directly from Easy Tables. If you already have a table created. Click on the table and then click the Edit script button to edit the files directly in Visual Studio Online.
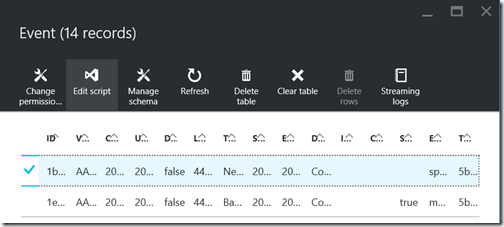
Here you can edit the files directly and they are saved immediately. I am going to start by create a table to store event information named Event. That means we need to create a file named event.js. Here is what the starting file looks like.
var azureMobileApps = require('azure-mobile-apps');
var table = azureMobileApps.table();
module.exports = table;
According to the documentation that is all that is required to get started with a new table. Now, you might be wondering where are the column names and types? Technically, they aren’t required. You see Easy Tables will create new columns on the fly when you make your first insert. This is great to try things out but not really what you want to do in a production environment. So I like to specify my own columns. You can use types such as string, date, boolean, and number. More complex types aren’t supported by the API. To create your columns put them in an object and assign to the columns property of table. Then be sure and set dynamicSchema to false so that it won’t create new columns on the fly. Set these values before calling module.exports.
table.columns = {
"title": "string",
"start_date": "date",
"end_date": "date",
"description": "string",
"image_url": "string",
"cost": "string",
"event_type": "string",
"send_notification": "boolean"
};
table.dynamicSchema = false;
Don’t worry about creating columns for things like and Id, created and modified dates, or even soft delete. Easy Tables will create those columns for you automatically.
Before Easy Tables goes and creates your back-end table, there are a few more steps. First, you need a corresponding .json file. In our case it would be Event.json. This contains some basic properties such as if soft-delete is enabled and whether certain operations require authentication. I found no documentation whatsoever that said this file was required. However, in the TodoItem samples out there on git hub the file was always there. Here is what it looks like.
{ "softDelete" : true, "autoIncrement": false, "read": { "access": "anonymous" }, "insert": { "access": "anonymous" }, "update": { "access": "anonymous" }, "delete": { "access": "anonymous" }, "undelete": { "access": "anonymous" }
}
If you want to authentication to be required, you can change the access for the corresponding operation to “authenticated”. However, we’ll cover that in a different post.
At this point, you should see an entry for your new table in the Easy Tables section of the Azure portal. However, the underlying SQL table does not exist yet. In fact, it doesn’t get created until you make your first API call to it. That part took me a while to figure out. Maybe there is a way to automate it getting created but in my experience it doesn’t happen until then. There is actually a parameter called seed that will let you put sample data into your table, but I have never successfully gotten it to work.
Calling the API using JavaScript
In my example, I am using Apache Cordova. It’s easy to get started with the Cordova SDK and Azure App Service. Just add the plugin and create a client object and start querying. Take a look at this post for more details. If you are using straight HTML / JavaScript, the code is basically the same as well. First, create a client object using the URL to your App Service. You can get this from the Azure Portal.
var client = new WindowsAzure.MobileServiceClient(azureMobileClientUrl);
Now, we can call getTable with the table name to get the event. Selecting data from the table is easy, just call .read() to get everything. It has a promise attached so that you can work with your results and errors accordingly.
var table = client.getTable('event');
return table.read()
.then(function (events) {
// do something with events
}, function (error) {
// do somethign with errors
});
If you want to filter your data just add a where clause. Each item you add to the collection will be treated as an “AND” operator. Note, that it is only simple equal comparisons though.
var table = client.getTable('event');
return table
.where({ id: myId})
.read()
.then(function (events) {
// do something with events
}, function (error) {
// do somethign with errors
});
You can also use .orderBy() to control the order of the data as well. It can be used in conjunction with the where clause if desired.
var table = client.getTable('event');
return table
.orderBy('start_date')
.read()
.then(function (events) {
// do something with events
}, function (error) {
// do somethign with errors
});
Making any of the above calls is enough to get your table created. You can then go to Server Explorer –> Azure –> SQL Databases and verify that the table was created. This is a great way to look and see what data is there as well.
Have a look at the SDK reference for inserting and updating data. You simply need to create an option with the matching column names and specify the values and call .insert() or .update() accordingly. Remember you don’t need to specify values for the Id or any of the other fields Easy Table creates. The response you get back will be the inserted or updated data.
var table = client.getTable('event');
return table.insert({
title: 'Event Title',
description: 'This is an event description',
start_date: new Date(),
end_date: new Date(),
event_type: 'Special Event',
send_notification: true
})
.then(function (response) {
}, function (error) {
});
If you need to delete data, the one thing I ran into is that it only works with the Id field. If you try to delete based on some other value, you’ll get an error. If you need to delete based on some other field, you will need to create your own API. We’ll cover that in another post.
var table = client.getTable('event');
table.del({ id: id })
.then(function () {
// deleted
}, function (error) {
// error
});
Mobile Apps in Azure App Service is an evolution of Azure Mobile Services. I often find there is more documentation on that since it’s been around longer. For example, take a look at this article on working with mobile data as it has a lot more examples.
Summary
Azure App Service Easy Tables make it super easy to get started creating a back-end for your mobile app. Give them a try and see what you can create. If you are looking for some samples, be sure and check out the Azure Mobile Apps repository in GitHub.