Microsoft Band is packed with sensors that let it track your workouts. However, one sensor you don’t hear about often is the Skin Temperature sensor. This sensor allows you to read the temperature in Celsius of your skin where your Band is. There aren’t any apps built-in to Microsoft Band that make use of it directly. However, as a developer you can take readings from that sensor from an app on your phone using the Microsoft Band SDK Preview. The SDK is very preliminary and you can only do a handful of functions from a phone including reading the sensors, creating tiles and sending notifications, and changing the theme and background image. It has a direct dependency on the app you deploy to your phone. You can’t actually build an app and deploy it so that it runs on Microsoft Band natively. This limits you quite a bit, but you can still do a few cool things.
For my example, I worked off the Sensors example project for Windows Phone 8.1 and made some customizations. The app consist of a simple button that initiates our connection to the Band and starts taking readings. You can customize the Sensors project as well or you can grab my copy from GitHub. You can get the SDK from nuget by using the following command. This will add the necessary project references.
Install-Package Microsoft.Band -Pre
Start by editing the XML of the Package.appxmanifest file and add the DeviceCapability section for Microsoft Band inside the Capabilities element.
<DeviceCapability Name="bluetooth.rfcomm" xmlns="http://schemas.microsoft.com/appx/2013/manifest">
<Device Id="any">
<!-- Used by the Microsoft Band SDK Preview -->
<Function Type="serviceId:A502CA9A-2BA5-413C-A4E0-13804E47B38F" />
<!-- Used by the Microsoft Band SDK Preview -->
<Function Type="serviceId:C742E1A2-6320-5ABC-9643-D206C677E580" />
</Device>
</DeviceCapability>
You also need to add the Proximity capability as well but you can do that through the UI.
Now, we’ll work on adding some functionality to MainPage.xaml. This consists mainly of a few TextBlocks and a Button. Clicking the button will invoke our code to connect to Band and take a reading. Create an event handling method for the button and mark it with async. Now, we first need to get the paired Bands to the phone. This assumes you have already paired your Band in the Bluetooth settings of the phone.
// Get the list of Microsoft Bands paired to the phone.
IBandInfo[] pairedBands = await BandClientManager.Instance.GetBandsAsync();
if (pairedBands.Length < 1)
{
this.textBlock.Text = "Band not paired.";
return;
}
To connect to Band we create an IBandClient in a using block.
using (IBandClient bandClient = await BandClientManager.Instance.ConnectAsync(pairedBands[0]))
{
}
Now we can read from the Skin Temperature sensor. To do this, we bind an event handling method to the ReadingChanged event of the SkinTemperature class. We’ll cover the event handling method just below.
bandClient.SensorManager.SkinTemperature.ReadingChanged += SkinTemperature_ReadingChanged;
Finally, we call StartReadingsAsync to start taking readings from Band. We follow it with a delay of a minute before calling StopReadingsAsync to stop taking readings.
await bandClient.SensorManager.SkinTemperature.StartReadingsAsync();
await Task.Delay(TimeSpan.FromMinutes(1));
await bandClient.SensorManager.SkinTemperature.StopReadingsAsync();
In the event handling method, the value of e has a type of BandSensorReadingEventArgs<IBandSkinTemperatureReading>. We can get the skin temperature in Celsius by using the SensorReading property. For us Americans, we can convert that to Fahrenheit. You know the formula right? C * (9/5) + 32. :). Using the Dispatcher, we then write the value of the reading to the UI.
private async void SkinTemperature_ReadingChanged(object sender, BandSensorReadingEventArgs<IBandSkinTemperatureReading> e)
{
IBandSkinTemperatureReading temperatureReading = e.SensorReading;
string text = string.Format("Temperature: {0}C", temperatureReading.Temperature);
await Dispatcher.RunAsync(CoreDispatcherPriority.Normal, () => { this.textBlock.Text = text; }).AsTask();
}
Now you are ready to run the program on your Windows Phone and see how hot you are! Plug your Windows Phone into your PC via the USB port and then debug the program and be sure you choose Device in the options. You can’t do this with the emulator.
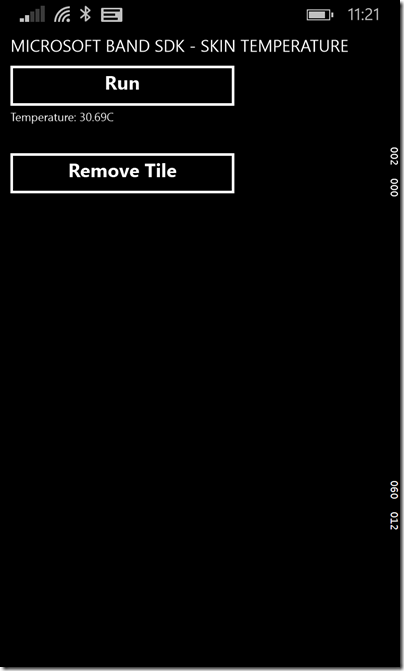
There you have it, my skin temperature was 30.69 Celsius (87.42 Fahrenheit). Now you might be thinking now, “ok, neat, but how do I see that information on my Band”. Well, you can push that information to your Band using a Tile. In fact, I’ve included that code in my repo on GitHub. However, you can only push information to your Band when the Windows Phone app is running. That’s not all that useful. I’ll cover tiles in a future post, but if you want to try it out for yourself, you can grab my code from GitHub. I would have included it today but I found that adding tiles so far doesn’t always work as expected. Maybe it will for you.
This example really has zero practical value but I expect that we’ll see more opened up in the API in future versions. If you have been interested in seeing what you can do with it as a developer, it’s worth checking out though. For more examples of working with Band across platforms, be sure and check out the published documentation.
Logic apps are a great new feature in Microsoft Azure that lets you connect to a variety of systems and create triggers and events. This allows you to reproduce some interesting light workflow functionality in an easy manner. It comes with connectors for common Microsoft products like SharePoint Online, Yammer, and BizTalk. However, it also includes connects to services like Twitter, SalesForce, SAP, and Dropbox. It even includes a connector for on-premises SharePoint 2013 servers although it requires some additional configuration.
As Logic apps are part of Azure, this is definitely a developer focused feature. I don’t see end users working with these directly.
Today, we’re going to look at expanding the Twitter / Dropbox example and expand that for use into SharePoint. That example stopped at only inserting the first tweet into a file on Dropbox. This example, will insert all tweets that have been returned from a user’s Mention timeline into a list on SharePoint Online.
First, I started by creating a simple custom list on my SharePoint Online (Office 365) site. I am just using the Title column as well as a custom column named TwitterAccount to capture who sent the tweet.
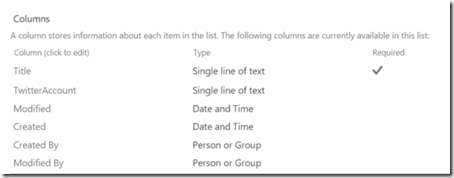
Like in the Twitter / Dropbox example, we need to create our connectors first. We’ll create the Twitter Connector first. Start by logging into the new Azure portal at http://portal.azure.com. Then click New, Developer Services, Azure Marketplace, API Apps.
Select Twitter Service and then click Create. Here you will be presented with some standard Azure settings that you will need to specify such as the App Service Plan, Resource Group, Subscription, and Location.
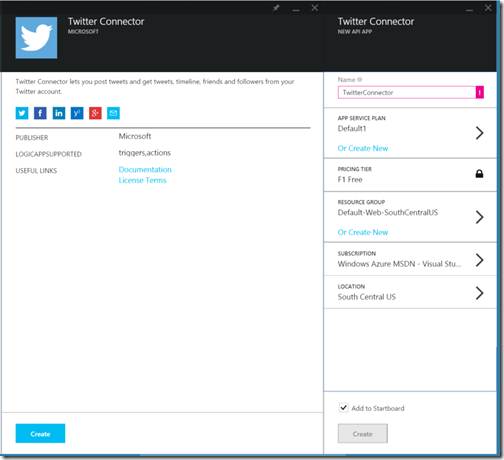
Now, we need to repeat the process for the SharePoint Online connector. Select the SharePoint Online connector out of the API Services list. Here you need to also specify the URL to your site as well as the relative URL to the list. For example, for my Tweets list I created, I specified a relative URL of Lists/Tweets.
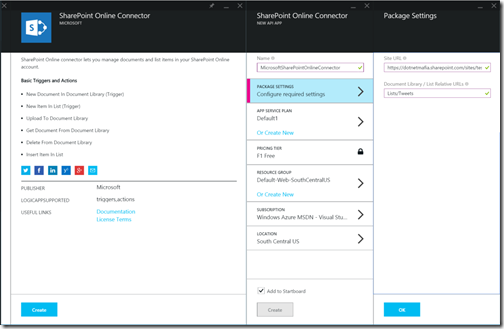
Now that we have created our connectors, we can get started with our Logic apps. Create a new one by choosing Logic app under the API Services list. If you can’t find any of these connectors or apps you can always just search for them. After you click Create, you will be prompted for some settings.
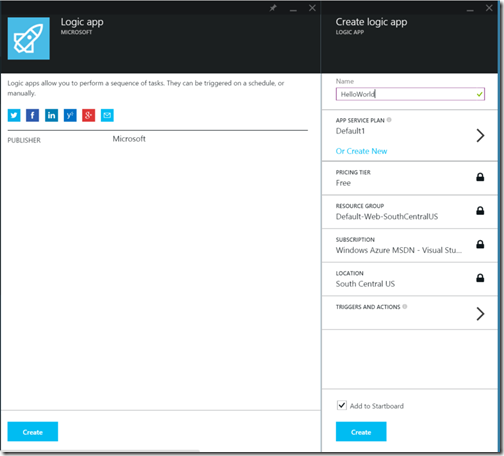
Give your app a name and then click Triggers and Actions to begin designing your app. The canvas will appear and you can begin designing your app.
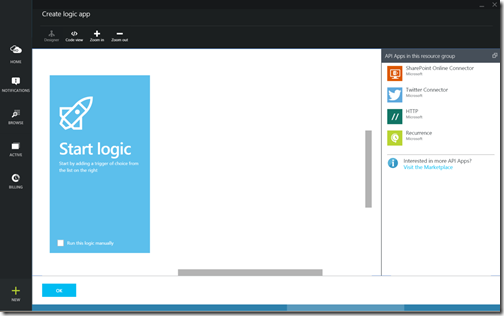
To get started you simply click on the connectors you want to use. The Twitter / Dropbox example starts with a recurrence connector to execute the task once an hour. I didn’t want to accidently leave this app running so I checked the box Run this logic manually.
Now we’ll click on the Twitter connector to pull data out of Twitter. The first time you do this you will be prompted for authentication by Twitter. Once you login, it will show a list of common actions. However, you can click the “…” to see more.
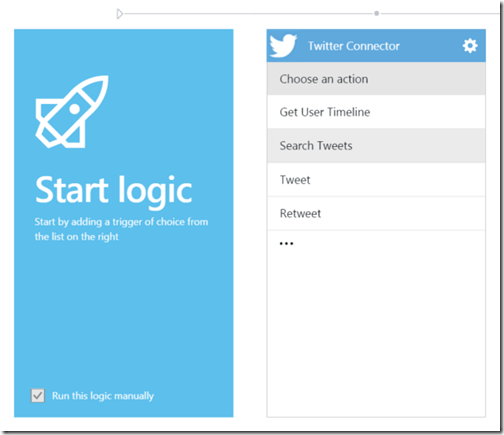
In our case, we want to select Get Mentions Timeline.
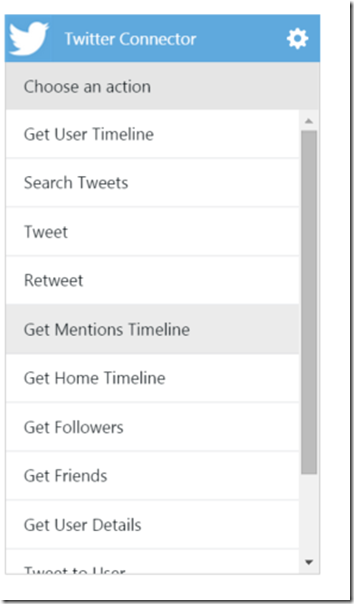
Now, we need to add our SharePoint Online Connector by clicking on it. It will also prompt you for authentication as well. It will then present you with common actions that you can preform on your list. It will mention your list directly by name. Choose Insert into <listname> to continue.
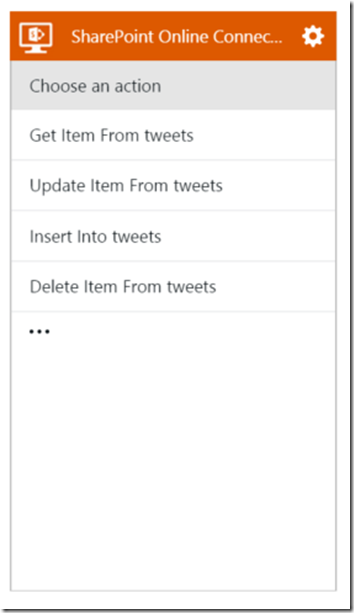
We’ll be able to start mapping our data to the columns in the SharePoint list. You can find the other fields by clicking on the “…” button.
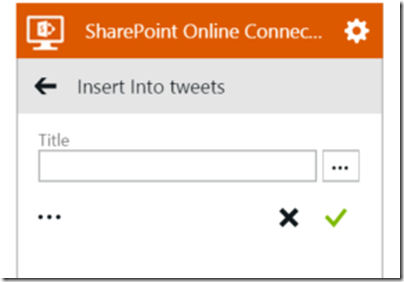
Don’t do the mapping yet though. In the online example, it only inserted the first list item. We’re going to implement repeating functionality to insert all of the list items. Click on the cogwheel and choose Repeat over a list.
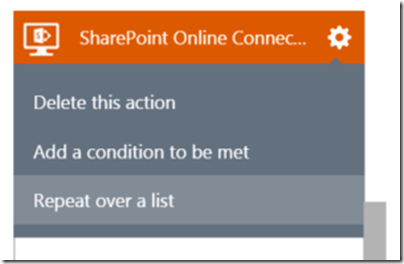
You can also specify conditions from this menu but we’ll cover that in the future. Now we want to specify the fields to use in our repeater. In the Repeat Field click the … icon next to it and choose Get Mentions Timeline. This will add the text @body(‘twitterconnector’) which is an internal syntax used by Logic apps. Now we want to write the Tweet Text to the Title field and the Tweeted By field to the TwitterAccount field. You can look at the options specified in the “…” menu but they don’t quite work right for repeaters. You can start by picking This Action but we still have a bit of work to do. It will add @repeatItem() to the box when you click it.
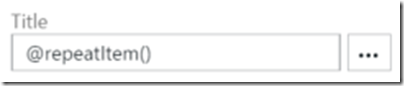
Now we need to specify the field name. We can actually infer this by looking at the Twitter Connector or by looking at the syntax it uses when we select a field from the “…” icon. Since this is a preview release, the selectors for these fields don’t always give the same results. Sometime if you select a field name it will do it correctly. Other times it gives us the syntax that only returns the value from the first item returned. Ultimately, I want to end up with a value of @repeatItem().TweetText for the Title field and a value of @repeatItem().Tweeted_By for the TwitterAccount field. Here’s what the final settings look like.
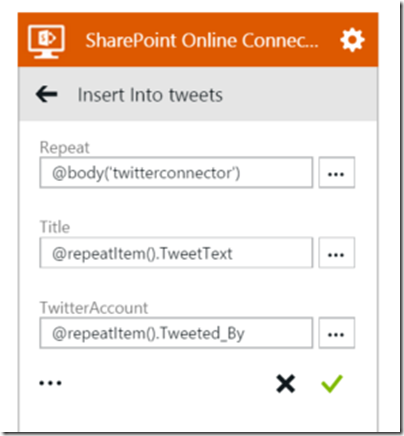
Click the checkbox to accept the values and then click Save at the top. Finally you want to click Create to begin creation of your Logic app. If you are going to make a lot of triggers and actions, I recommend you click Create first before you create them. This will allow you save your progress as you work on things. Nothing will be saved until you have created the Logic app itself first.
Now are ready to manually run the Logic app manually. Click the Run Now button at the top to begin. You’ll see the progress in the Operations section at the bottom.
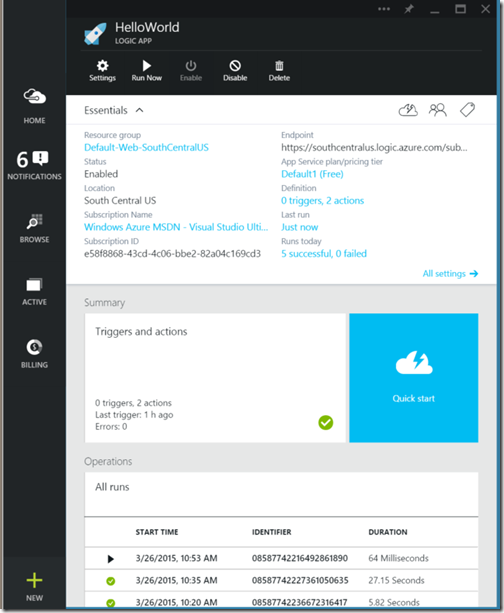
Go back to the list on your SharePoint site to verify that the functionality worked. You can see the list of tweets that mentioned me have been populated.
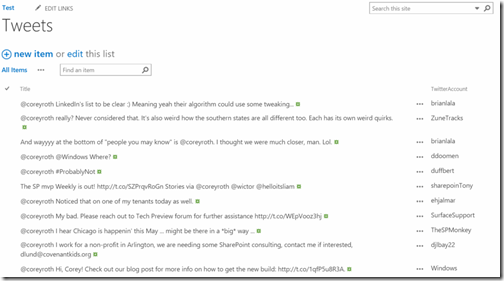
I noticed they didn’t necessarily come in the right order so it might be a good idea to bring in the date column as well. When I made a schema change to my list earlier I had to recreate the SharePoint Online Connector for it to show up. There might be a way to refresh it though.
Logic Apps are an interesting technology with a lot of promise. It makes some scenarios rather easy to implement in a short time. I’ll continue to work with the technology to get a feel for what it can do and what the limitations are. Try it out for yourself and see what you can do.
I’ve done quite a bit of work with the client-side People Picker in SharePoint-hosted apps lately. I find that while it is relatively easy to get set up, I found it was quite easy to break. I have found that it tended to work better in Office 365 than it does in on-premises SharePoint 2013. I ran into two common issues. First, I found that after you did a query for users, you could not actually select the user. The second related issue is that you could select the user, but the textbox would remain empty and not show the user’s name.
In my troubleshooting of the issue, I found that the behavior differed based on a variety of things including what user was using the page, how you authenticated, and which browser you were using. When I was experiencing issues, I found that administrator users never had a problem. That figures. Low-privilege users would not be able to get the control to work. Then I discovered that it would work for these users if they were not auto logged into the site with Internet Explorer. Here, I am referring to the setting Automatic logon with current user name and password. If the user typed in their credentials manually, it would actually work. Quite strange.
After further investigation, I discovered that access to the spMgr object deep in clientpeoplepicker.js was coming back as undefined. This turns out to be in a block of code that shows presence information for the user. I found that if I disabled presence, it fixed the issue for users in Internet Explorer. To disable presence, set ShowUserPresence to false on the SPClientPeoplePicker object. Do this right before your call to SPClientPeoplePicker_InitStandaloneControlWrapper.
SPClientPeoplePicker.ShowUserPresence = false;
This resolved my issues in Internet Explorer. However, Chrome was displaying some erratic behavior. Sometimes the People Picker would work, but most of the time it still wouldn’t. Although this technique worked for some browsers, I don’t think it was the final solution. I found the final solution also in the debugger. I reported the following error in clienttemplates.js:
Strings is not defined.
The odd thing is that the minified version of strings.js was actually included on the page automatically but it didn’t work for some reason. Strings.js is the JavaScript file that contains all of the multilingual string resources for your current culture. I included a hard reference to the US English version of the strings.js file in the 1033 folder.
<script type="text/javascript" src="/_layouts/15/1033/strings.js"></script>
Once I did that, all of my People Picker issues were resolved. You may want to correct culture code for the languages you use.