July 2013 - Posts
Quite some time ago, I wrote about how to set default column values using C#. However, I find it useful to work with them using PowerShell quite often. The reason being, I often use this as a utility to work set default column values on document libraries or folders in bulk. I often do this as an exercise of adding metadata to document libraries that didn’t have it previously. I was doing this recently for a client and I wanted to make use of managed metadata columns and found that setting a default column value of this type is not straight forward. This is no surprise though, since anytime you deal with managed metadata columns, nothing is as it seems. Let me show you what you need to know.
Start a new PowerShell script file (.ps1). The first thing we need to do is add some assemblies for referencing both the term store and the default column values.
[System.Reflection.Assembly]::LoadWithPartialName("Microsoft.SharePoint.Taxonomy")
[System.Reflection.Assembly]::LoadWithPartialName("Microsoft.Office.DocumentManagement")
To do this, we first need to get a reference to the actual term object. We need this because of the way we set the default column value. To do this we’re going to need a site collection object as well. We can do this with standard PowerShell commands. Specify the entire path to the subsite that you need because we are just going to use SPSite.OpenWeb() to get our SP-Web object for later.
$site = Get-SPSite http://server/sitecollection
Take a look at my term set as we’ll use the values here in our PowerShell commands to get the term we want. In this case, we are going to use the selected term, Invoice.
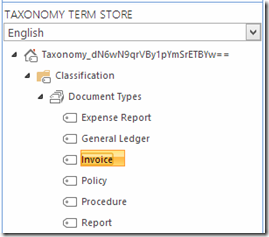
I am going to then issue the following statements to get the term.
$session = New-Object Microsoft.SharePoint.Taxonomy.TaxonomySession($site)
$termStore = $session.TermStores[0]
$group = $termStore.Groups["Classification"]
$termSet = $group.TermSets["Document Types"]
$terms = $termSet.GetAllTerms()
$term = $terms | ?{$_.Name –eq “Invoice”}
First, we need to get a new TaxonomySession using the SPSite object we got earlier. We then open the default term store using an indexer of 0. Once we have a TermStore object, we can get the group we need, Classification. Then we get the Document Types TermSet using an indexer. At this point, we can call GetAllTerms() to return all terms in the term set. Note, that this can be an expensive operation, but we really have no choice. Lastly, we use a standard PowerShell technique to compare the list of terms and select one by name. That leaves us with the Term object we need.
One we have the term we need, we need to get a reference to the document library on the site we are working with. Start by getting an SPWeb object.
$web = $site.OpenWeb()
Now, we are just going to get the list we need by name using an indexer.
$list = $web.Lists["Documents"]
At this point, we are ready to set our default column values. This code will actually look similar to my previous blog post. Start by getting a MetadataDefaults object. You’ll need to pass in the SPList object we just created.
$columnDefaults = New-Object Microsoft.Office.DocumentManagement.MetadataDefaults($list)
Now we use the SetFieldDefault() method to create our defaults. It requires a SPFolder object, the internal name of the SPField (not display name), and the value itself. If we were working with a Single line of text field, it would look something like this.
$columnDefaults.SetFieldDefault($list.RootFolder, "MyTextField", “MyTextValue”)
However, we are working with Managed Metadata, so if you try to assign the value like that, you’ll get the following error message.
The given value for a taxonomy field was not formatted in the required <int>;#<label>|<guid> format.
If you are not familiar with that format, let me explain. The first value is the LCID code. A lot of you use English so likely a value of 1033 will work. If that isn’t your default language, you probably know your LCID code or you can go look it up. Then, the value it expects for <label> is the text value of the term, for that we just use $term.Name. Lastly, we need the GUID of the term, so for that we use $term.Id. This gives us a command that looks like this.
$columnDefaults.SetFieldDefault($list.RootFolder, "MyManagedMetadataField", "1033;#" + $term.Name + "|" + $term.Id)
Finally, with any SharePoint construct, we need to call Update() to commit our changes.
$columnDefaults.Update();
If you got everything correct, that should work for you. You’ll want to add you error handling of course, but hopefully, this is enough to get you started.
For SharePoint 2010, I wrote a post that covered some typical service accounts that you might need along with suggested names. I wanted to update the list for SharePoint 2013 as I get asked these questions a lot by people new to installing things. This list is of course open to debate but this is typically what I personally am going to start with when setting up a new multi-server SharePoint 2013 farm. Did I miss anything? Do you have any suggestions? Leave a comment!
Here is the list I typically start with. I have included a brief description of what the account is used for and the required permissions.
Account | Permissions | Description |
sp_Setup | - SQL Server – dbcreator and securityadmin roles
- Local administrator on SharePoint servers
| - This account is used to perform the initial install and configuration of SharePoint.
- Technically not a service account
|
sp_Farm | - SQL Server – dbcreator and securityadmin roles
- Allow log on locally
- Log on as a service
| - SharePoint farm account specified in SharePoint Configuration Wizard
- This account also will have local administrator privileges when provisioning User Profile Synchronization
|
sp_PortalAppPool | | - Application pool account for main SharePoint web application
- Could also just be called sp_AppPool or spAppPool + <PortNumber>
|
sp_ServiceAppPool | | - Application pool account for web application hosting service applications
|
sp_MySitesAppPool | | - Application pool account for My Sites web application
|
sp_UserProfileSync | | - Account used to synchronize user profiles from Active Directory
|
sp_Search | | - Account used for running Search Service
|
sp_SearchCrawl | - Full Read on each web application
| - This account is used by search when crawling
- This account must not have local administrator permission or SharePoint administrator permissions
|
sp_SuperUser | | - Used for caching on publishing sites.
|
sp_SuperReader | - Full-read permission in User Policy for Web Application
| - Used for caching on publishing sites.
|
sp_Workflow | - SQL Server – dbcreator and securityadmin roles
- Log on as a service
- Allow log as locally
| - Login with this account when installing Workflow Manager
- Be sure to specify this account user@domain format.
- Used for Workflow Manager and Service Bus services
- Should not be the same account as farm account
|
Note for some of the permissions such as “Log on as a batch job” or “Log on as a service”, you will not have to set unless you are in an environment that specifically locks these down.
Some SQL accounts that you will likely use:
Account | Description |
sp_SQLService | - Service account for SQL Server.
|
sp_SQLAgent | - SQL Server Agent account.
|
sp_SSAS | - Service account for SQL Server Analysis Services.
|
sp_SSRS | - Service account for SQL Server Reporting Services.
|
This is a decent list to get you started. For reasons why you might want this many accounts be sure and check out my SharePoint 2010 post on the same topic. That post covers why you want multiple accounts and reminds you that you should use different accounts for production environments. Let me know what you think and I’ll post updated to the list! Thanks!
I’ve suffered through my share of SharePoint upgrade / migration projects now and I have really changed the way I look at them. Often when you get exposed to a new system, the lack of governance has led to a total mess with site sprawl as far as the eye can see. The architect in me makes me want to fix everything: upgrade, fix the IA, classify content, re-brand, you name it. You might be tempted to do it all at once and maybe even use a third-party tool that promises to migrate things from location to another seamlessly. These tools can prove handy, but don’t fall into the trap though. The risk is too high and the experience can be jolting. Let me explain my thoughts.
For me it’s all about the user and judging what success is. You want to minimize the impact to the user. The more you change at once, the higher the risk. The more changes you make, the more likely you will fail and then your users will revolt. Think about it. Just changing user interfaces can be jarring. Every version of SharePoint looks a lot different. Now, you want to tell the users that all of their files are going to have new URLs too? Now it might make sense in your mind because on change is easier than two. However, I’ve tried it before, it doesn’t go well. You end up troubleshooting upgrade issues as well as a heap of other issues because links are broken (yes, I know there are tools that help with that).
Upgrade First
Why do I like upgrading first? Because it can be viewed as a (relatively) quick win. Most SharePoint farms aren’t easy to upgrade, but sometimes they are. You throw your solution packages on the new farm, attach the content databases and you are good to go. I’m over simplifying it I know, but I think approach makes sense. With SharePoint 2013 and site collection upgrades, it makes it much less painful to upgrade a site one at a time. Since sites can run in SharePoint 2010 mode during a transition period. If things go well, you can actually transition your users over to 2010 mode on the new 2013 servers and then decommission the old servers.
Switching SharePoint versions requires us to make changes to branding every time. A lot of times, companies use this as an opportunity to redo the home page, relaunch the Intranet or make other changes. Don’t do that. Again, too much change. Honestly, your users may appreciate the fact that they can drag and drop files into a document library more than the fact that you’ve come up with a flashy new news rotator on the home page. They just want to be able to find the content they need to do their jobs efficiently. That’s why I am saying this is not the time to rearrange sites and start tagging content. So what do we do about the branding though? Chances are you don’t want to completely lose your corporate brand when you hit that upgrade site collection button. My recommendation is build a new master page but it keep pretty similar to the one you had from the previous version of SharePoint. This may seem like a wasted effort since nothing you still have to rebuild your master page from scratch. It’s a painful process I know, but it makes sense in the end. Deploy your new master page to the 2013 version of the site and start upgrading your site collections.
What is great about this approach is your users are using the new version of SharePoint much faster. This gives you a win. What happens when you decide to change your information architecture or branding is then you have to get multiple departments involved marketing, corporate communications, compliance, records management, legal, and whomever else things they have a say. Add those departments to your upgrade project and you’ve just extended your upgrade project by six months.
Fix the Information Architecture
Now, that you have your new site running on the latest version of SharePoint and your users are excited about the change, you can start thinking about governance enforcement and information architecture. This approach allows you to site down with a department, determine their needs, and explain to them that you need to move their site, split it into two, or whatever needed to make it fit into the current organization properly. As a consultant, this is an excellent opportunity to meet more business users and potentially even drum up new work. Users will often open up to you when you sit down with them and tell you what frustrates them about their current process. They especially get excited when you show them how some new feature can tackle some issue that they had with the last version of SharePoint.
Changing Information Architecture doesn’t always mean you are moving sites around either. What’s important is getting the navigation right. It needs to be clear and intuitive to the users. Users generally have some idea how the company is organized and they are going to look for things in that manner. At the same time, you are going to find content you want to highlight such as policies, forms, and more that should be in the navigation as well. There are plenty of ideas out there on how to arrange your navigation, you have to find the one that is right for your organization. Just remember, it’s about making it as painless as possible for your users to find the information they need.
If you need to move or consolidate sites, SharePoint doesn’t exactly make it easy with the tools that are included out-of-the-box. I urge you to proceed with caution when evaluating migration tools with ISVs. There are a lot of tools out there and they all have their plusses and minuses. You need to keep in mind whether the tool properly maintains authors and dates when moving. You need to especially tread carefully, if you need to move a site with workflows. The things that tools have to do to move workflows is a bit unsettling so I always tread carefully. However, the tools are getting better and better so you may run into less issues than I have in the past.
Depending on the size of the company, chances are you can’t make information architecture changes overnight. You’ll have to move things and update navigation in batches. Of course there are infrastructure considerations as well. Your sites need to be arranged in content databases accordingly to scale for growth. We could write a whole series of posts on arranging sites, navigation, and planning for scalability though. Just from these few paragraphs, you may be thinking about the amount of time and planning this can require. The point I want to make here today is that your IA changes should come after you do your upgrade. If you are still waiting to decide on these changes, your upgrade, still hasn’t occurred.
Re-launch the Site
What I mean by this is now is when you take the opportunity to redesign the home page, implement new features, change the branding, and more. This is the time when you get your marketing and corporate communications people to drum up excitement about the site. After all it’s a lot more fun to celebrate a new site that has new features tailored to the organization’s employees than it is to celebrate that SharePoint is running a new version. Your IT department might be celebrating that, but the rest of your company could probably care less unless there is just some specific feature that changes one of their lives.
What do you think? This subject is widely open for debate and there are lots of ways to approach it. What has worked for you? What hasn’t? Share your story in the comments below.
Now, you might be asking why not just use a tool to migrate and reshape the IA at the same time. I’ve tried that and it worked for some stuff very well. However, if you have a site that is tricky and the tool struggles with, then people start asking where there files are or why did they lose content on a page or worse. Effectively this doubles the amount of testing you need to do. You may thing you are saving time, but it just has too much risk. Upgrade first, then change your Information Architecture. Your users will appreciate it.