Configuring Site Collection Search Settings with PowerShell
Posted
Wednesday, December 21, 2011 10:28 AM
by
CoreyRoth
I’m always looking for a way to automate things and configuring the search settings of a site collection is no exception. I’ve talked about the Search Settings page before and how important it is for configuring contextual search and the scope drop down on your master page. Wouldn’t it be nice to be able to automate these changes instead of having to manually set it on every site collection?
To configure these settings, we first must understand where they are. It turns out that they can be found in the AllProperties collection of the root SPWeb object in a site collection. On a new site collection or on one where you have never configured the search settings, no values will exist in the property bag for search. However, one you go to the search settings page and click save, it will write the values into the property bag. As you may know, the search settings page has three configurable fields. These values on the search settings page map to the values below in the property bag.
Setting | Property Bag Name |
Site Collection Search Center | SRCH_ENH_FTR_URL |
Site Collection Search Dropdown Mode | SRCH_SITE_DROPDOWN_MODE |
Site Collection Search Results Page | SRCH_TRAGET_RESULTS_PAGE |
Now what goes in each value? SRCH_ENH_FTR_URL typically takes a relative URL (i.e. /search/pages). However, it can take full URL as well. SRCH_SITE_DROPDOWN_MODE requires you pass a special string based upon what you want the drop down to display (i.e.: Show Scopes, Don’t Show Scopes, Default to s parameter, etc.) How do you know what string to pass? Well the easiest way to determine this is to go to the Search Settings page of the site collection and view source. Search for the drop down mode you want and you will find the string in the value parameter of an option element. Here is what it looks like.
<option selected="selected" value="HideScopeDD_DefaultContextual">Do not show scopes
dropdown, and default to contextual scope</option>
<option value="HideScopeDD">Do not show scopes dropdown, and default to target results
page</option>
<option value="ShowDD">Show scopes dropdown</option>
<option value="ShowDD_DefaultURL">Show, and default to 's' URL parameter</option>
<option value="ShowDD_DefaultContextual">Show and default to contextual scope</option>
<option value="ShowDD_NoContextual">Show, do not include contextual scopes</option>
<option value="ShowDD_NoContextual_DefaultURL">Show, do not include contextual scopes,
and default to 's' URL parameter</option>
For example, I typically use the option Show and default to contextual scope, so I would choose ShowDD_DefaultContextual.
The last value, SRCH_TRAGET_RESULTS_PAGE cracks me up. Note how the word target is misspelled. It looks like something slipped past QA there. This value specifies the URL to the contextual search page. Typically, I change this to use the URL to my search center as I have mentioned before. Anyhow, if you want to set this value be sure and misspell it.
Now that we know what values we need to set, how do we put it all together with PowerShell? It’s pretty simple. First we need access to the SPWeb object of the root web of the site collection we want to change. You can use Get-SPSite first to do this, but if you know the URL, then you can just use Get-SPWeb. Either way you do it, get yourself an SPWeb object. Here is an example:
$web = Get-SPWeb http://server/sitecollection
If you have the right permissions, you should get a blank prompt back without error. If you get an access denied error, then you need to go make use of Add-SPShellAdmin.
Now that you have the SPWeb object, you can see if any values have been set for search by using the AllProperties collection.
$web.AllProperties
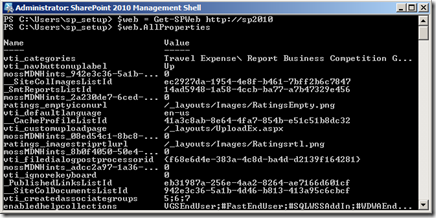
In my case, no properties have been set yet. Now I can set my values. You can set one or more of these in your script.
$web.AllProperties["SRCH_ENH_FTR_URL"] = "/search/pages";
$web.AllProperties["SRCH_SITE_DROPDOWN_MODE"] = "ShowDD_DefaultContextual";
$web.AllProperties["SRCH_TRAGET_RESULTS_PAGE"] = "http://server/search/pages/results.aspx";
After you set your values, don’t forget to call .Update() just like you would when working directly with the object model.
$web.Update();
Executing the script should just return you to the PowerShell prompt if everything worked. You can verify your settings, by looking in the AllProperties collection. For example. Just make sure you get a new instance of the SPWeb object first or you won’t see the changes.
$web = Get-SPWeb http://server/sitecollection
$web.AllProperties["SRCH_ENH_FTR_URL"]

As you can see it’s really simple. Now what if you wanted to set these settings on all site collections?
$webApp = Get-SPWebApplication http://server;
$siteCollections = $webApp | Get-SPSite –limit all;
$siteCollections | ForEach-Object {
$web = $_.RootWeb
$web.AllProperties["SRCH_ENH_FTR_URL"] = "/search/pages";
$web.AllProperties["SRCH_SITE_DROPDOWN_MODE"] = "ShowDD_DefaultContextual";
$web.AllProperties["SRCH_TRAGET_RESULTS_PAGE"] = "http://server/search/pages/results.aspx";
$web.Update();
}
Try that out and see if that works for you.